Internet Explorer Aborting AJAX Requests : FIXED
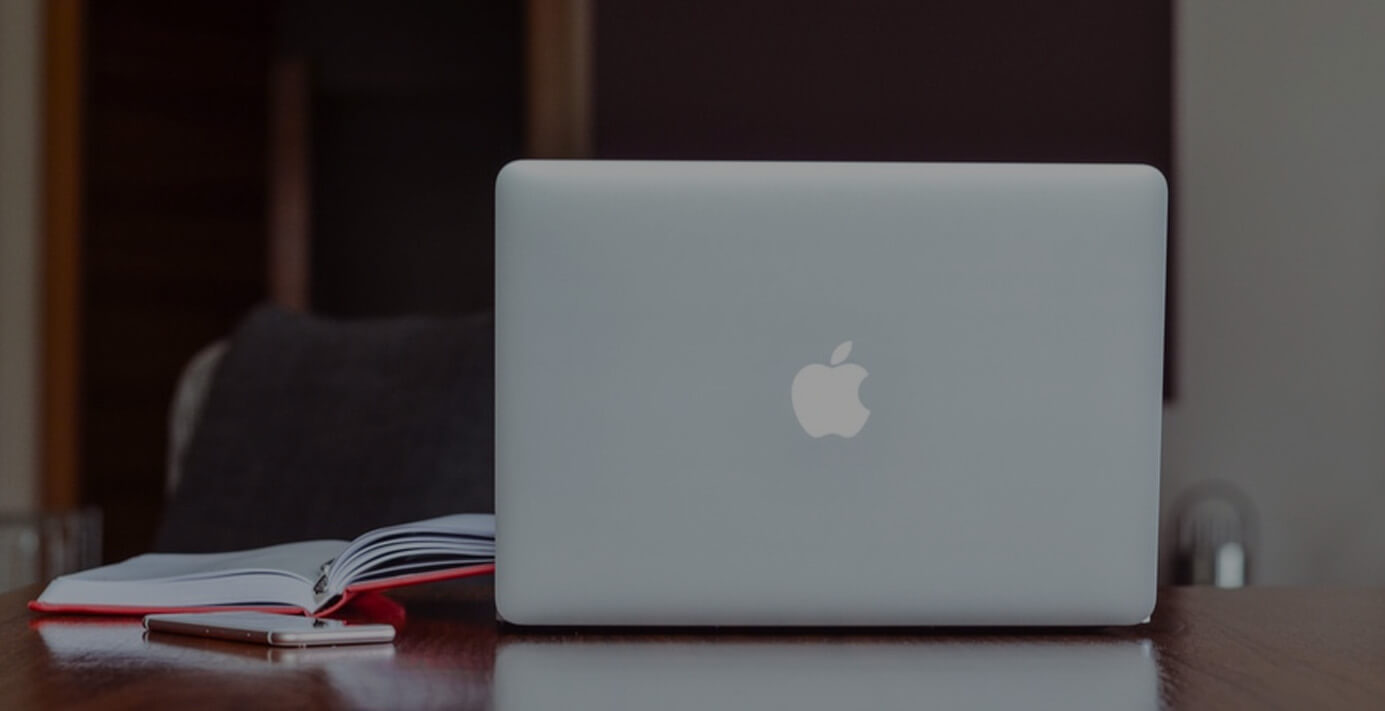
I've written previously on how to handle AJAX requests for Internet Explorer but recently we came across a strange issue where the requests were being aborted by IE before the response was finished being delivered. Using Fiddler and Firebug, we were able to see that the request was being made properly, and even the response was coming back properly, but at some point, IE would simply stop accepting the data if it was not a very short response.
The problem was even more perplexing because it did not happen every time and it did not happen the same in all versions of IE, in fact IE 9 had more difficulty than IE 7.
You might be familiar with the typical way of making an AJAX request using XDR for Internet Explorer. It would generally look like the following:
//USING JQUERY if ($.browser.msie && window.XDomainRequest) { // Use Microsoft XDR var xdr = new XDomainRequest(); xdr.open("get", "someurl"); xdr.onload = function () { var JSON = $.parseJSON(xdr.responseText); if (JSON == null || typeof (JSON) == 'undefined') { JSON = $.parseJSON(data.firstChild.textContent); } processData(JSON); }; xdr.send(); }
The process goes like this:
- Create the XDomainRequest object
- Define the request type (GET,POST) and the request path (URL) in .open()
- Define an event handler to capture the response in .onload()
- Send the request using .send()
Depending on what you are returning, this is usually all you need.If you run into the problem that we were having, you need to do a little more to fix it.
The Solution
The problem has to do with IE timing out the request even though data is being transmitted. By defining some additional event handlers and specifying a timeout value of 0, IE will not abort the request prematurely. Your mileage may vary but for us the solution was to define the following handlers as empty:
xdr.onprogress = function () { }; xdr.ontimeout = function () { }; xdr.onerror = function () { };
Then wrap the send() function in a timeout declaration:
setTimeout(function () { xdr.send(); }, 0);
Producing the final resulting XDR call of:
if ($.browser.msie && window.XDomainRequest) { // Use Microsoft XDR var xdr = new XDomainRequest(); xdr.open("get", "someurl"); xdr.onload = function () { var JSON = $.parseJSON(xdr.responseText); if (JSON == null || typeof (JSON) == 'undefined') { JSON = $.parseJSON(data.firstChild.textContent); } processData(JSON); }; xdr.onprogress = function(){ }; xdr.ontimeout = function(){ }; xdr.onerror = function () { }; setTimeout(function(){ xdr.send(); }, 0); }
26 Comments
Leave a Reply
Meet the Author
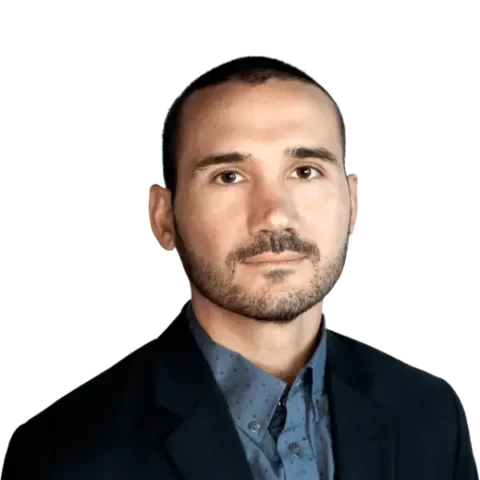
Matthew Mombrea
Matt is our Chief Technology Officer and one of the founders of our agency. He started Cypress North in 2010 with Greg Finn, and now leads our Buffalo office. As the head of our development team, Matt oversees all of our technical strategy and software and systems design efforts.
With more than 19 years of software engineering experience, Matt has the knowledge and expertise to help our clients find solutions that will solve their problems and help them reach their goals. He is dedicated to doing things the right way and finding the right custom solution for each client, all while accounting for long-term maintainability and technical debt.
Matt is a Buffalo native and graduated from St. Bonaventure University, where he studied computer science.
When he’s not at work, Matt enjoys spending time with his kids and his dog. He also likes to golf, snowboard, and roast coffee.
This doesn't work for a POST json operation. If I'm looking to use json data to get a request in a subdomain. Currently M$'s craptacular browsers inhibit such transfer as x-domain. The XDR seems like the only alternative to jsonp, but I'm not seeing it working.
Andrew,
Check these posts to see if the help your issue:
http://www.cypressnorth.com/blog/programming/cross-domain-ajax-request-with-json-response-for-iefirefoxchrome-safari-jquery/
http://www.cypressnorth.com/blog/programming/cross-domain-ajax-request-with-xml-response-for-iefirefoxchrome-safari-jquery/
Thanks. This helped greatly on a get json XDR that was mysteriously barfing in IE9.
It's pathetic that IE requires one to jump through such odd hoops.
Bravo. This saved me a lot of pain and anguish.
I an not a computer buff could you ive a step by step procedure
on how to fix this "aborting" problem I have Vista basics.
Thanks this helps me
Thanks, just what I needed!
I had given up on a solution until I found this. Now our IE9 users can use SVG, and not Flash! Awesome!
Just to save anyone else that has to do this with IE some grief. You have to set the ontimeout, onprogress, onload, onerror, and timeout values AFTER the xdr.open method. Also, on this line:
setTimeout(function () {
xdr.send();
}, 0);
I had to change it to:
setTimeout(function () {
xdr.send();
}, 500);
To get it to work. My theory is that initializing this XDomainRequest object takes some time depending on the resources of the client box. 500ms was a good value for us to get a variety of host machines to work consistently.
Thank you so much for this!
You are a god among men. I spent hours trying to figure out this goofy intermittent IE9 problem.
Thanks a lot for this help !
This is not working for me in IE7 it works in all other versions of IE
if($.browser.msie && window.XDomainRequest) {
var xdr = new XDomainRequest();
xdr.open("get", ajaxStatusUrl);
setTimeout(function() {
xdr.send();
}, 500);
xdr.onload = function() {
var JSON = $.parseJSON(xdr.responseText);
if(JSON == null || typeof (JSON) == 'undefined') {
JSON = $.parseJSON(data.firstChild,textContent);
}
processData(JSON);
};
xdr.onprogress = function() {};
xdr.ontimeout = function() {};
xdr.onerror = function() {
alert('error');
};
} else {
$.ajax({
type: "GET",
url: ajaxStatusUrl,
processData: true,
dataType: "json",
success: function(data) {
processData(data);
}
});
}
});
Also fiddler is not showing a request being made, it says XDomainRequest obejct is undefined
Varun,
This could be due to a different issue with IE caching GET requests. Try changing your request to a POST instead and see if that takes care of the problem.
Or you can leave it as a GET and append the current time ticks to the ajaxStatusUrl variable. See this post for more details:
http://www.itworld.com/development/303295/ajax-requests-not-executing-or-updating-internet-explorer-solution
Hi Matthew thanks for your quick response, I have tried all the methods mentioned in the link however for IE7 it's still not making the ajax request at all here is my updated code
$.ajaxSetup({ cache: false });
if($.browser.msie && window.XDomainRequest) {
var xdr = new XDomainRequest();
xdr.open("post", ajaxStatusUrl+"?buster="+ new Date().getTime());
setTimeout(function() {
xdr.send();
}, 500);
xdr.onload = function() {
var JSON = $.parseJSON(xdr.responseText);
if(JSON == null || typeof (JSON) == 'undefined') {
JSON = $.parseJSON(data.firstChild,textContent);
}
processData(JSON);
};
xdr.onprogress = function() {};
xdr.ontimeout = function() {};
xdr.onerror = function() {
alert('error');
};
} else {
$.ajax({
type: "POST",
url: ajaxStatusUrl+"?buster="+ new Date().getTime(),
processData: true,
dataType: "json",
success: function(data) {
processData(data);
}
});
}
});
I also changed the server endpoint to accept post instead of get and set the response header to
header("Cache-Control: no-cache, no-store"); header("Expires: Sat, 26 Jul 1997 05:00:00 GMT"); // Date in the past
so i managed to get the ajax request working in IE with this code change
if($.browser.msie) {
if(window.XDomainRequest == undefined) {
var xdr = new ActiveXObject('Msxml2.XMLHTTP');
} else {
var xdr = new XDomainRequest();
}
xdr.open("post", ajaxStatusUrl+"?buster="+ new Date().getTime());
setTimeout(function() {
xdr.send();
}, 1000);
xdr.onload = function() {
var JSON = $.parseJSON(xdr.responseText);
if(JSON == null || typeof (JSON) == 'undefined') {
JSON = $.parseJSON(data.firstChild,textContent);
}
processData(JSON);
};
xdr.onprogress = function() {};
xdr.ontimeout = function() {};
xdr.onerror = function() {
alert('error');
};
} else {
$.ajax({
type: "POST",
url: ajaxStatusUrl+"?buster="+ new Date().getTime(),
processData: true,
dataType: "json",
success: function(data) {
processData(data);
}
});
}
});
however the onload function xdr.onload is throwing an error saying object doesn't support this property or method
var xdr = new ActiveXObject('Microsoft.XMLHTTP');
incase of IE 7
New UPDATE
I got the code to work locally
// Check if the browser is IE
if($.browser.msie) {
var xdr;
// Check if the browser is IE7 or IE 10+
if(window.XDomainRequest == undefined && window.XMLHttpRequest) {
xdr = new XMLHttpRequest();
xdr.onreadystatechange = function() {
if(xdr.readyState == 4 && xdr.status == 200) {
var JSON = $.parseJSON(xdr.responseText);
if(JSON == null || typeof (JSON) == 'undefined') {
JSON = $.parseJSON(data.firstChild,textContent);
}
processData(JSON);
}
}
} else { // IE8, IE9
xdr = new XDomainRequest();
xdr.onload = function() {
var JSON = $.parseJSON(xdr.responseText);
if(JSON == null || typeof (JSON) == 'undefined') {
JSON = $.parseJSON(data.firstChild,textContent);
}
processData(JSON);
};
xdr.onprogress = function() {};
xdr.ontimeout = function() {};
xdr.onerror = function() {
alert('error');
};
}
xdr.open("post", ajaxStatusUrl+"?buster="+ new Date().getTime());
setTimeout(function() {
xdr.send();
}, 1000);
} else { // all other browsers
$.ajax({
type: "POST",
url: ajaxStatusUrl+"?buster="+ new Date().getTime(),
processData: true,
dataType: "json",
success: function(data) {
processData(data);
}
});
}
});
But on production IE 7 is giving access denied
I tried your code for a POST call to ASP.NET Webservices asmx and your code for IE8 and IE9 always return error 500.
Here is my code which gives 'JSON Undefined' in IE 8, Can you please let me know how to convert this below AJAX code using XDR
var dataToSend =
{
ClientId: selClientId
};
$.ajax({
url: '',
contentType: 'application/json; charset=utf-8',
type: "POST",
data: JSON.stringify(dataToSend),
dataType: "json",
success: function (data) {
document.getElementById("dvClientName").innerHTML = data[0];
}
}
});
It sounds like you need to include the json2.js polyfill on your page. https://github.com/douglascrockford/JSON-js/blob/master/json2.js. This will add support for JSON.stringify and JSON.parse in older browsers.
Thanks for the help. This was an odd problem to say the least and this does seem to work. Just out of curiosity, do you have any idea what the actual problem is and why this fixes it?
I don't know why this works but it works! So thank you a lot, you saved a lot of my time!
Really, really, thank you. I had no idea how you worked this out, and I dread to think how much time you lost 🙁 but it solved my IE9 AJAX woes!!
I generally don't comment on sites. But I just had to here. I spent an entire day figuring out the solution and you sir, solved it for me. 3 in the morning and I feel so effing happy. Thanks a lot 🙂
Made my day. Thanks.
Is there a solution using jquery ajax ?